ProductDetails
The ProductDetails
component renders the interface for creating new products and viewing product information. It displays details of an existing product item and allows a user to edit the product.
Preview
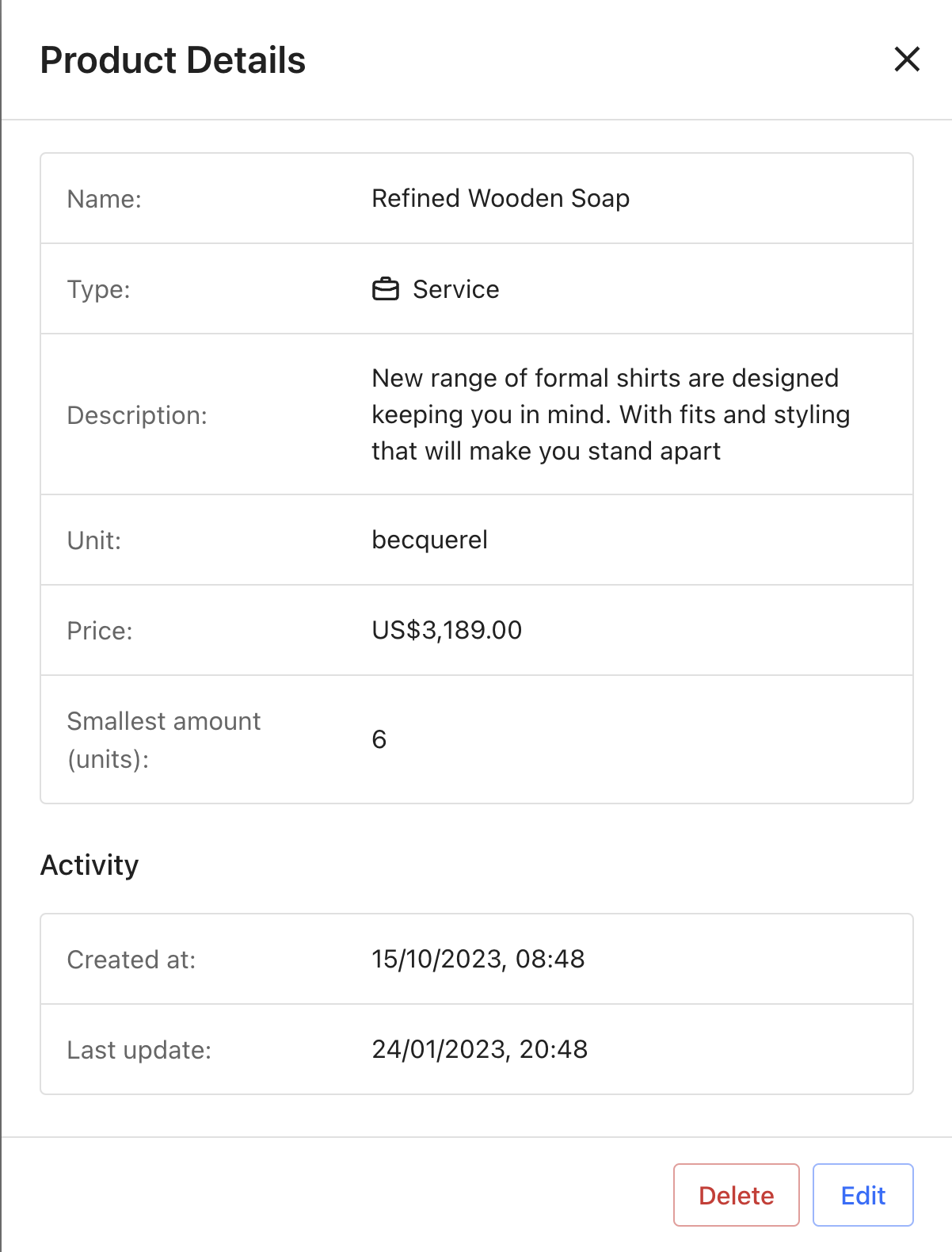
ProductDetails
component preview.
Usage
You can use the ProductDetails
component to create, view, edit, and delete details of an existing product. To create a new product, use the ProductDetails
component without any props as shown:
import { Dialog, ProductDetails } from "@monite/sdk-react"
...
// TODO: This component must be rendered within the MoniteProvider wrapper
const ProductDetailsPage = () => {
return (
<Dialog open alignDialog="right">
<ProductDetails />
</Dialog>
);
};
To view details of an existing product, use the ProductDetails
component with the id
prop as shown:
import { Dialog, ProductDetails } from "@monite/sdk-react"
...
// TODO: This component must be rendered within the MoniteProvider wrapper
const ProductDetailsPage = () => {
return (
<Dialog open alignDialog="right">
<ProductDetails id="PRODUCT_ID" />
</Dialog>
);
};
To edit details of an existing product, you must provide the product ID via the id
prop and set the initialView
prop to Edit mode.
import { Dialog, ProductDetails } from "@monite/sdk-react"
...
// TODO: This component must be rendered within the MoniteProvider wrapper
const ProductDetailsPage = () => {
return (
<Dialog open alignDialog="right">
<ProductDetails
id="PRODUCT_ID"
initialView="edit"
/>
</Dialog>
);
};
Props
Prop | Type | Description |
---|---|---|
defaultValues | object | This object is used to set default form values when creating a new product. |
id | string | This is a required prop that accepts the UUID of the product to be displayed. |
initialView | ("edit"| "read") | The initialView prop determines whether the component is rendered in Read or Edit mode. Defaults to read . |
onCreated | function | This callback is triggered when a new product is created. |
onDeleted | function | This callback is triggered after a product is deleted. |
onEdit | function | This callback is triggered when a product is edited. |
onUpdate | function | This callback is triggered when a product information is updated. |
Updated about 2 months ago