ReceivablesTable component
Overview
ReceivablesTable
is a React component that displays all accounts receivable documents - invoices, quotes, and credit notes - created by an entity. The component supports searching, filtering, and pagination between different receivables.
Permissions
To access this component, the entity user must have read
permissions for the receivable
object. For more information, see List of permissions.
Preview
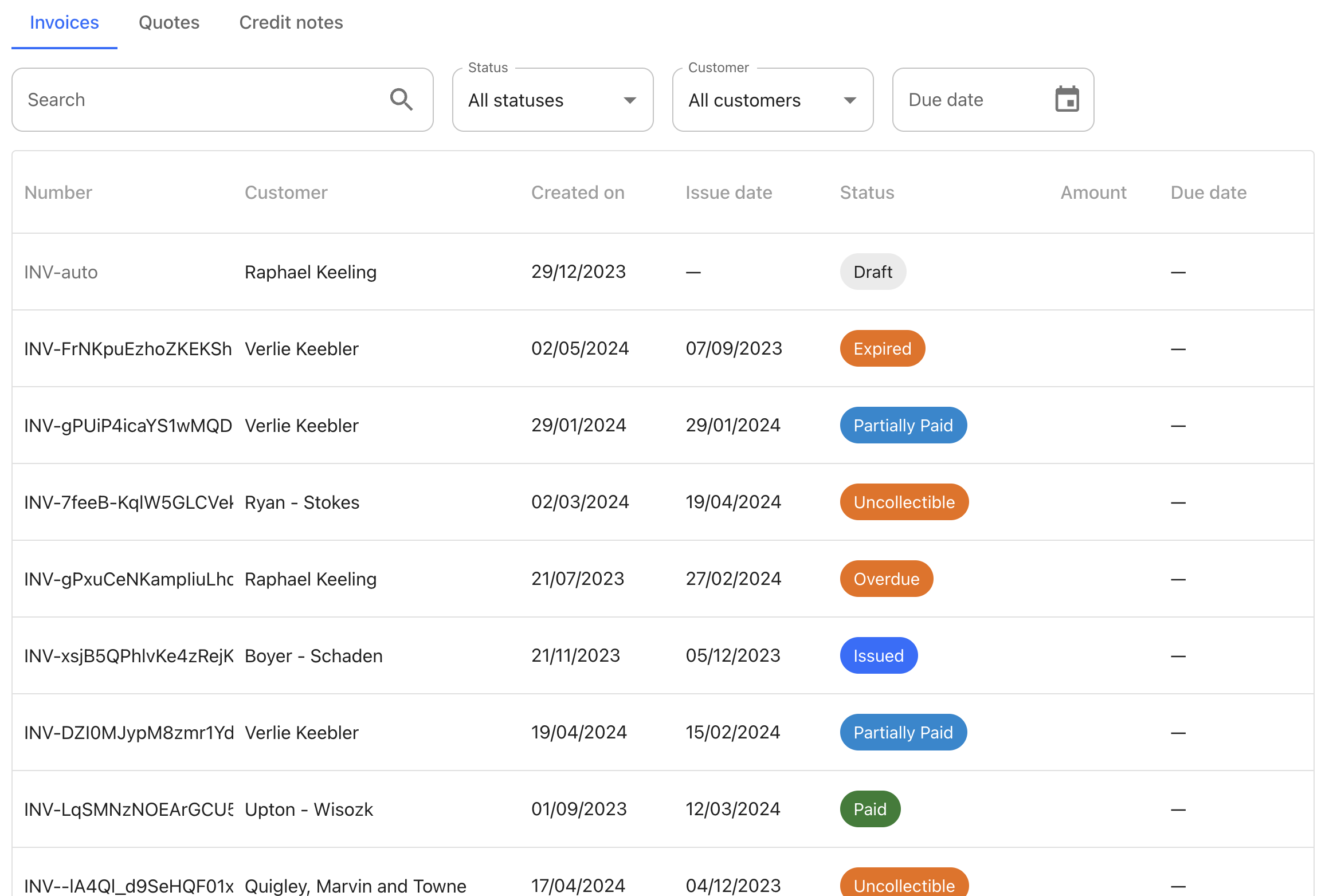
Usage
Use the ReceivablesTable
component in your application as follows:
Props
This callback takes the identifier of the clicked row as a parameter and is triggered when a receivables table row is clicked.
This callback is triggered any time the component’s tab is changed.
Gets or sets the currently active tab in the ReceivablesTable
component. The value is the tab’s 0-based index from left to right.
When using the default tabs, possible values are:
- 0 - Invoices tab
- 1 - Quotes tab
- 2 - Credit notes tab
The list of displayed tabs. Each tab object defines the data query used to populate the table and the search filters displayed above the table. The default tabs are Invoices, Quotes, and Credit notes. The default filters for each tab are the document number (document_id
), status, counterpart, plus the due date filter for invoices. See Customize the tabs for more information and examples.
Customize the tabs
You can use the tabs
prop to fully customize the tabs displayed in the ReceivablesTable
component. For example, you can:
- hide some of the default tabs,
- display different document statuses in different tabs (for example, display draft and paid invoices separately),
- adjust the API queries used to fetch documents for each tab.
The tabs
value has the following structure: