Authentication
Learn how to authenticate your API requests to the Monite API platform.
Monite API provides you with industry-standard security controls to connect to its platform, submit, store and retrieve data from Monite data servers, and perform various business operations with this data.
API security key concepts
The Monite API platform enforces certain principles to maintain the integrity and security of data:
- Secure Data Transmission: API requests to the Monite API platform must be made via HTTPS. Any calls attempted over plain HTTP will not be successful.
- Bearer Token Authentication: All API requests must authenticate with a
Bearer
token, generated by Monite from the supplied Client ID and Secret values. This method is in line with, and compliant with OAuth 2.0, the accepted protocol for API authorization. - Role-Based Access Control (RBAC): Upon receiving an API request made with a specific token, Monite verifies whether the token bearer has permissions to perform the requested operation(s). This is guided by the RBAC model.
- Resource Ownership: In Monite, resources are linked to specific entities (as defined in Monite account structure). To access resources owned by a specific entity, the API call must include the unique entity ID value in the
x-monite-entity-id
HTTP header.
Client ID and client secret
Monite’s token mechanism aligns with the OAuth 2.0 standard, enabling generation of short-lived tokens. These tokens have an adjustable lifespan and are considered more secure than longer-lived API keys. They also mitigate damage from potential leaks.
Before any tokens can be generated for the Monite API, partner developers need to log into their account on the Monite Partner Portal. Here, developers generate a pair of Client ID and Client Secret values.
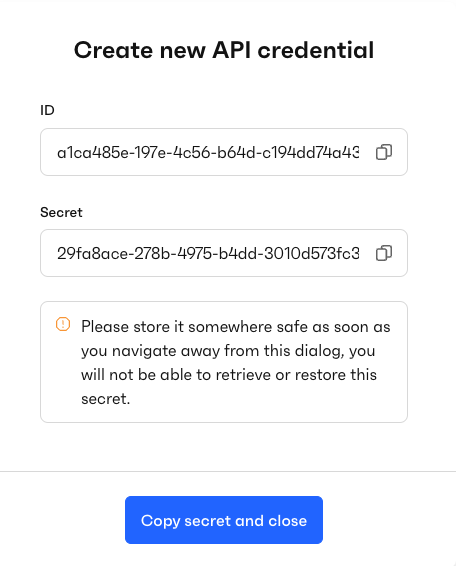
The client secret must be stored securely as it will not be visible again after its creation.
Monite staff are unable to retrieve your secret, so losing it necessitates generating a new one.
Creating tokens
To create a new token for accessing the Monite API, first you need to call POST /auth/token
with the request body containing the client_id
and client_secret
values you obtained in the previous step. Additionally, you need to specify the grant_type
, which can have one of the following values:
client_credentials
- a standard OAuth 2.0 grant type for backend-to-backend communication. We refer to this token as a partner-level token.entity_user
- a custom grant type used by Monite. We refer to this token as an entity-user token.
The /auth/token
endpoint does not require any authentication headers, which is in line with the OAuth 2.0 standard. At the same time, we strongly recommend that you pass the x-monite-version
HTTP header to specify the API version in use. This is important because the payload structure of /auth/token
might change in future API versions.
The successful 200 OK
response contains the access_token
and its validity time (in seconds):
Revoking tokens
Tokens generated within the Monite API platform have an auto-expiry based on the specified expires_in
value (in seconds). Nevertheless, in certain cases you may want to explicitly revoke an active token, such as when a user logs out from your application.
To revoke a token, call POST /auth/revoke
with a request body containing the client_id
, client_secret
, and the token
itself:
A 200 OK
response indicates that the specified token has been revoked successfully.
Partner token
A partner token is a token with the highest privileges within the Monite API platform. To generate a partner token, call POST /auth/token
with grant_type
set to client_credentials
:
When to use partner tokens:
- To perform some operations that are allowed for a partner token only. For example, all operations with the
/entities
collection (such as creating, reading, and updating entities) or changing a partner’s/settings
are allowed for partner tokens, but not for entity user tokens. - If you make all access control and permission checks using your own permissions model and want to override Monite’s internal RBAC system. In this case, we recommend that you use the partner token in the backend-to-backend communication only.
Entity user token
An entity user token is a specific token issued for an individual entity user. Its permissions are defined by the role associated with the user. These tokens enhance security because they rely on the Monite RBAC system, allowing actions to be directly linked to specific users within your platform.
To generate an entity user token, call POST /auth/token
with grant_type
set to entity_user
, and specify the ID of this user in the entity_user_id
request field:
A successful 200 OK
response contains the entity user-level access_token
and its validity time (in seconds):
If an entity user has a custom role assigned to it, the list of permissions will be taken from this role. If no role is assigned to this user, the role will be taken from the default user role configured for the entity.
For example, a role that includes permissions to add, view, update, and delete entity users would look like this:
Permission types
When you create a role, you need to specify which permissions should be applied to specific actions on certain objects that exist in the Monite platform.
The following permission types are available:
not_allowed
(default) - the specified action is not allowed.allowed
- the specified action is always allowed.allowed_for_own
- the specified action is allowed to be performed only on the objects created by this entity user.
List of permissions
Below is the list of all permissions available in the Monite RBAC system:
Choosing your security model
When integrating your application or platform with Monite, you have three security models to choose from based on your requirements for user roles and rights management:
- Monite-managed security (recommended)
- Partner-managed security
- Hybrid security
The specifics of each model are explained below.
Monite-managed security model (recommended)
With this model, Access Control Lists (ACLs) and access tokens manage the access to the Monite API. Entities map all their users into Monite and set the ACL rights for these users within Monite. Partners then issue entity-user tokens for subsequent API calls and apply ACL access control to these tokens. Advantages of this model include:
- Expedited integration process and quicker time to market.
- ACL is managed by Monite.
- Monite maintains comprehensive records of entity users and roles as set by the partner.
Partner-managed security model
In this model, partners manage security outside of Monite and make all API calls using the partner-level token. This provides root access to entity data, without the need to provide any information about user logins and access rights. The potential downsides of this approach include:
- Increased complexity in implementation and higher risk of data incidents.
- Partners are required to populate optional API fields and store user IDs assigned by Monite in order to map actions to specific users.
Hybrid security model
This model keeps Monite informed of entity user IDs and login data, while partners manage the ACL externally. Monite stores user information for reference purposes only. While this provides compatibility with the partner’s system, it does not provide a significant advantage over either Monite-managed or Partner-managed security models.