SDK customization
Learn how to customize the components on the Monite React SDK.
Overview
The Monite components use the default settings by default. However, there are scenarios where you can to customize this settings to match the needs of your business.
The monite.ts
file
The monite.ts
file exports an object that contains several configuration options. These options provide the foundation for controlling the settings within the Monite SDK.
Customizable components
MonitePayableTable
Add tabs with filters
You can select additional tabs to be added to the payables table, which will display the results of specific filters. See an example with tabs/filters for unpaid, schedules, and paid payables:
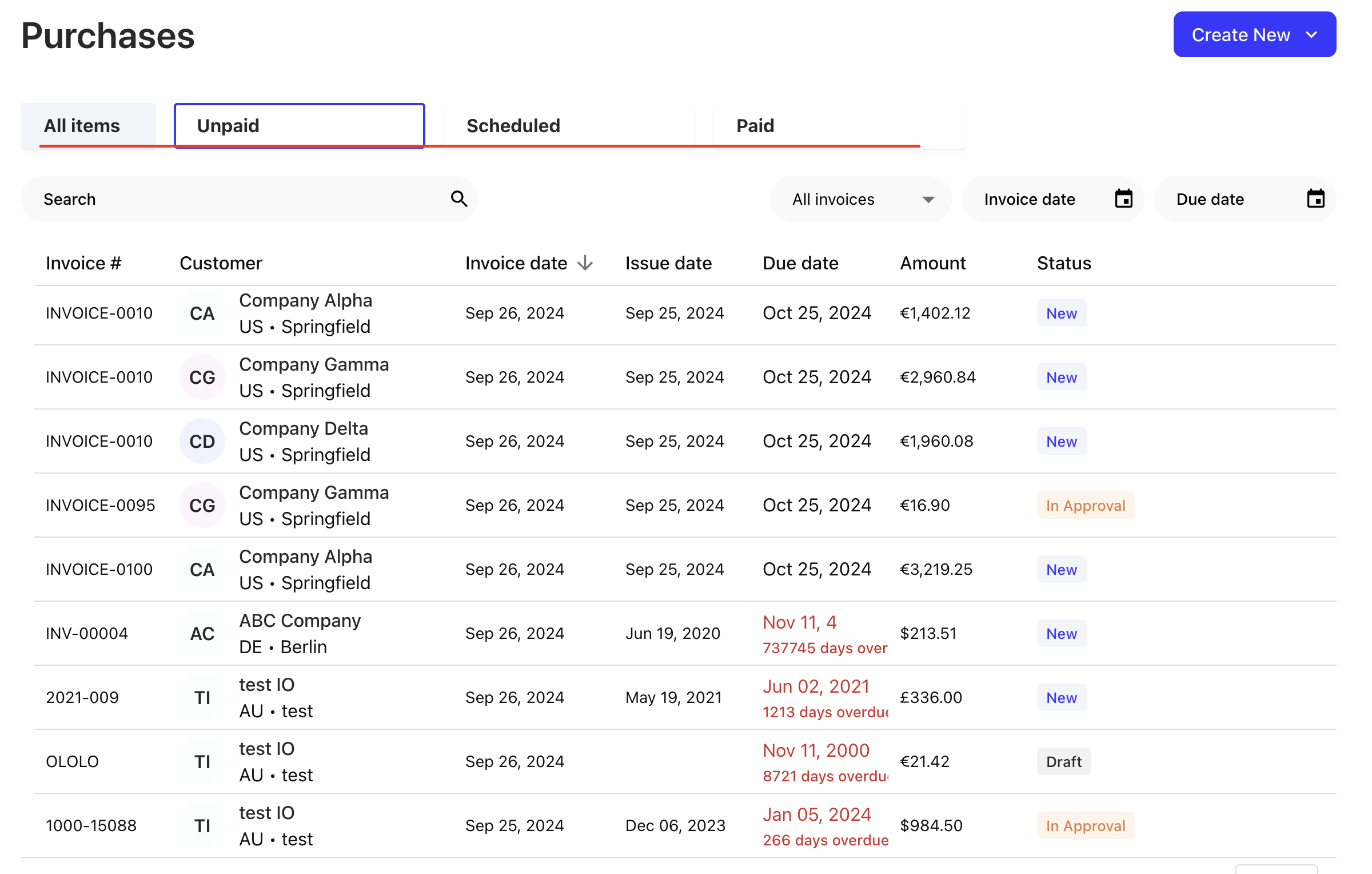
The required fields must be informed in the summaryCardFilters
field. Here is an example:
Some fields of the code explained:
Unpaid
,Scheduled
,Paid
- The labels of the tab.status__in
,tag_ids
- The filters of the tab.
See the GET /payables
endpoint for all possible filters.
MonitePayableDetails
Set fields to be mandatory for review after OCR
You can select which fields of the Payable are mandatory to be filled after the OCR process. The fields that were not scanned will be marked as required with a red asterisk:
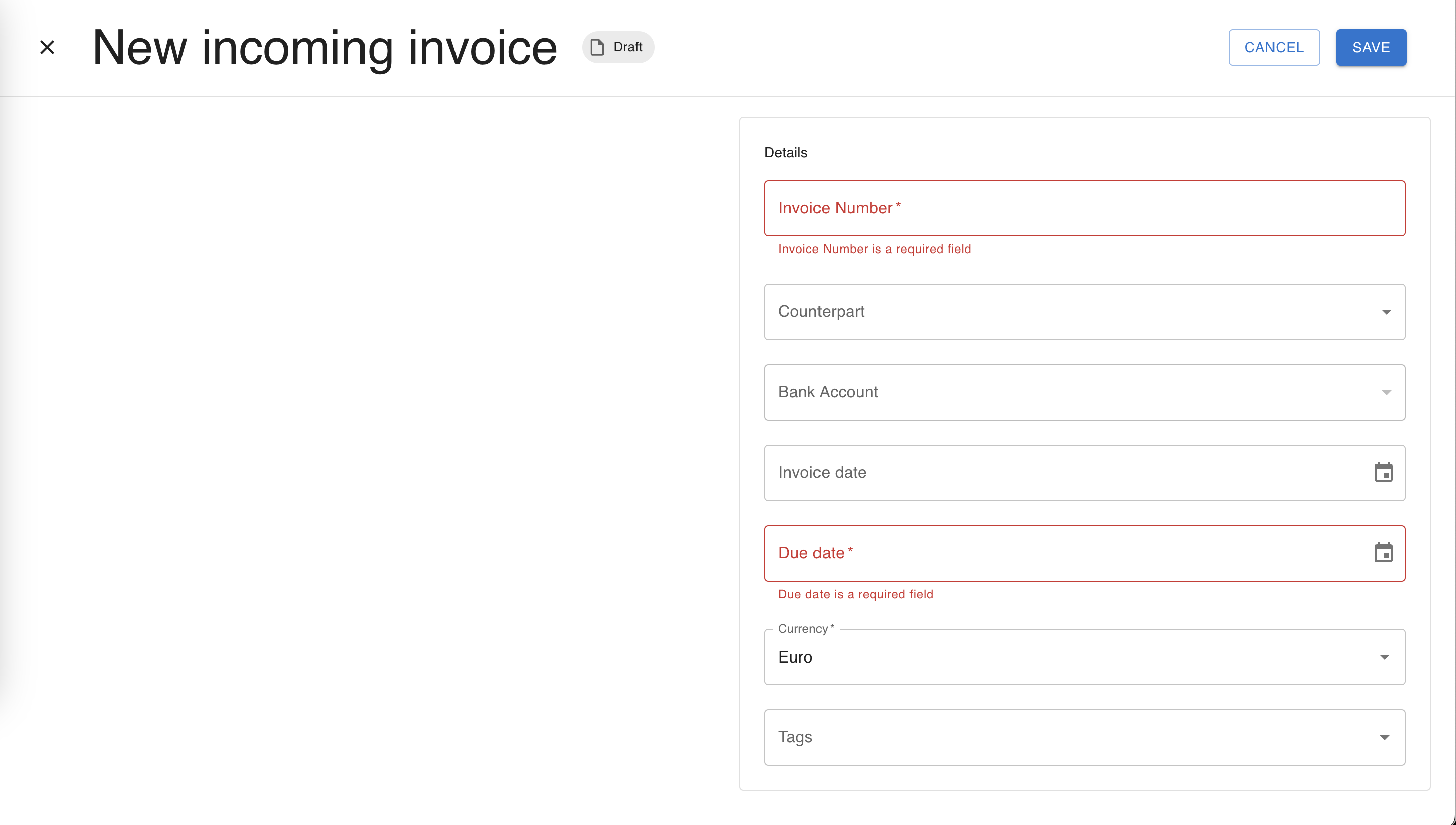
The required fields must be informed in the ocrRequiredFields
field. Here is an example: