Drop-in quick start
Get started with Monite's Drop-in web component.
The fastest way to embed Monite's UI into your web application is to utilize the Drop-in library, which contains pre-built JavaScript web components that can be integrated into almost all types of web apps.
To learn how this can be done, follow the steps in this tutorial.
Before you begin
Before proceeding with this tutorial, make sure you completed the steps in the Step 1: Get your credentials and Step 2: Implement server side guides.
1. Open your web app or create a new one
If you already have a web application, open it in your favourite IDE.
Alternatively, you can create a new app from scratch using one of the popular bootstrapping frameworks. For example, create an empty React application using create-react-app:
npx create-react-app my-app
Then you can open this folder in VS Code, explore its source code or type npm start
in a terminal to see the app in action.
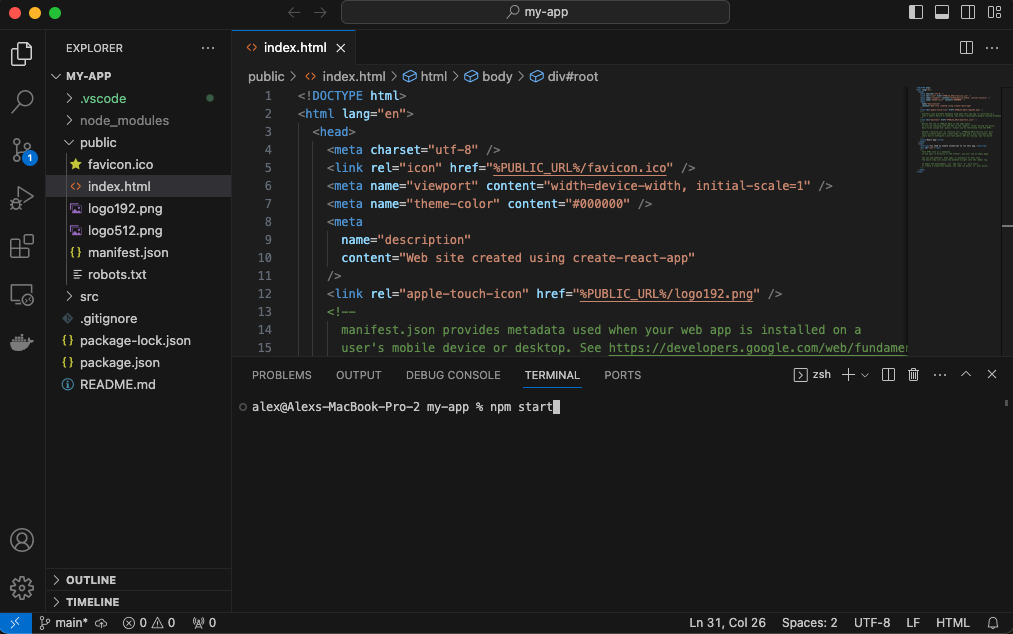
2. Add monite to your app
The fastest way to add monite to your app is to download monite-app.js
from a CDN. Doing this requires no "build step", as the process does not require module bundlers.
For this, locate the index.html
file in your web app file and include the following script
tag within your HTML file's body
:
<script type="module" async src="https://sdk-demo.sandbox.monite.com/monite-app.js"></script>
Alternatively, you can also use NPM or Yarn to install monite as a package:
npm install @monite/sdk-drop-in
yarn add @monite/sdk-drop-in
After installation via NPM or Yarn, you must import the SDK to use Monite's Drop-in components in your application:
<script type="module">
import "@monite/sdk-drop-in";
</script>
useEffect(() => {
import("@monite/sdk-drop-in");
}, []);
3. Embed the monite-app
element
monite-app
elementThe monite-app
element is a custom HTML element that contains the UI and configuration for Monite's Drop-in components.
Add the monite-app
element to your application and include the required attributes:
<monite-app
api-url="https://api.sandbox.monite.com/v1"
entity-id="ENTITY_ID"
component="payables"
basename="/"
></monite-app>
return (
<div className="App">
<div
dangerouslySetInnerHTML={{
__html: `
<monite-app
basename="/"
entity-id="ENTITY_ID"
api-url="https://api.sandbox.monite.com/v1"
>
</monite-app>
`,
}}
/>
</div>
);
Here, the basename
parameter should be set to the base URL for all routes in your application, but you can leave it empty in this tutorial.
Note that the entity-id
parameter here should be automatically set to the entity ID you generated in Step 2: Implement server side.
4: Set up user authentication
Every entity user of the Monite app should authorize themselves with a mechanism that is used in your web application. When a user is authorized by you, you should also issue an entity user token, as described in the previous Step 2: Implement server side.
If you already implemented a backend part that is capable of issuing entity user tokens, then write a function that retrieves this token and add it to the <script slot="fetch-token">
of the monite-app
.
However, if you haven't implemented the backend part yet but can already generate an entity and entity user token, you can also just make a POST
request to the /auth/token
endpoint directly from this script, as shown below:
<script slot="fetch-token">
async function fetchToken() {
const request = {
grant_type: "entity_user",
entity_user_id: "ENTITY_USER_ID",
client_id: "CLIENT_ID",
client_secret: "CLIENT_SECRET",
};
const response = await fetch(
"https://api.sandbox.monite.com/v1/auth/token",
{
method: "POST",
headers: {
"Content-Type": "application/json",
"x-monite-version": "2024-01-31",
},
body: JSON.stringify(request),
}
);
return await response.json();
}
</script>
While the code about should work perfectly fine for the demo purposes, the best practice is always to generate Monite tokens from your server side code.
5: Review the code
Finally, you can compare your resulting code with our template to make sure that everything is correct:
<!DOCTYPE html>
<html lang="en">
<body>
<script type="module">
import "@monite/sdk-drop-in";
</script>
<monite-app
entity-id="ENTITY_ID"
api-url="https://api.sandbox.monite.com/v1"
component="payables"
basename="/"
>
<script slot="fetch-token">
async function fetchToken() {
const request = {
grant_type: "entity_user",
entity_user_id: "ENTITY_USER_ID",
client_id: "CLIENT_ID",
client_secret: "CLIENT_SECRET",
};
const response = await fetch(
"https://api.sandbox.monite.com/v1/auth/token",
{
method: "POST",
headers: {
"Content-Type": "application/json",
"x-monite-version": "2024-01-31",
},
body: JSON.stringify(request),
}
);
return await response.json();
}
</script>
</monite-app>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<body>
<script
type="module"
async
src="https://sdk-demo.sandbox.monite.com/monite-app.js"
></script>
<monite-app
entity-id="ENTITY_ID"
api-url="https://api.sandbox.monite.com/v1"
component="payables"
basename="/"
>
<script slot="fetch-token">
async function fetchToken() {
const request = {
grant_type: "entity_user",
entity_user_id: "ENTITY_USER_ID",
client_id: "CLIENT_ID",
client_secret: "CLIENT_SECRET",
};
const response = await fetch(
"https://api.sandbox.monite.com/v1/auth/token",
{
method: "POST",
headers: {
"Content-Type": "application/json",
"x-monite-version": "2024-01-31",
},
body: JSON.stringify(request),
}
);
return await response.json();
}
</script>
</monite-app>
</body>
</html>
return (
<div className="App">
<div
dangerouslySetInnerHTML={{
__html: `
<script slot="fetch-token">
async function fetchToken() {
const request = {
grant_type: "entity_user",
entity_user_id: "ENTITY_USER_ID",
client_id: "CLIENT_ID",
client_secret: "CLIENT_SECRET",
};
const response = await fetch(
"https://api.sandbox.monite.com/v1/auth/token",
{
method: "POST",
headers: {
"Content-Type": "application/json",
"x-monite-version": "2024-01-31",
},
body: JSON.stringify(request),
}
);
return await response.json();
}
</script>
<monite-app
entity-id="ENTITY_ID"
api-url="https://api.sandbox.monite.com/v1"
component="payables"
basename="/"
></monite-app>
`,
}}
/>
</div>
);
(Optional) Add theming
You can customize the theme of the monite-app
component. To include theming, define the object that describes the custom themes within a script
tag in your file. The script
tag that contains your custom theme is assigned an slot
attribute with a value of theme
as shown:
<!-- Create new theme JSON -->
<script slot="theme" type="application/json">
{
"typography": {
"fontFamily": "Comic Sans MS, Comic Sans, cursive, monospace",
"h2": {
"fontSize": "26px",
"fontWeight": "500"
}
}
}
</script>
For more information on customizing Monite Drop-in UI, see Theming and customization.
Next, insert the script
into in the monite-app
element as shown:
<monite-app entity-id="ENTITY_ID">
<!-- Embed JSON into the monite-app element -->
<script slot="theme" type="application/json">
{
"typography": {
"fontFamily": "Comic Sans MS, Comic Sans, cursive, monospace",
"h2": {
"fontSize": "26px",
"fontWeight": "500"
}
}
}
</script>
</monite-app>
(Optional) Add localization
You can customize the locale of the monite-app
component renderings. To include localization, define the object that describes the localization within a script
tag in your file. The script
tag that contains your custom theme is assigned an slot
attribute with a value of locale
:
<!-- Create new locale JSON -->
<script slot="locale" type="application/json">
{
"code": "de-DE",
"messages": { "Counterparts": "Suppliers" }
}
</script>
The
currencyLocale
property must adhere to the BCP-47 language tag standard. For more information on including localization in Monite's Drop-in library, see Localization.
Next, insert the script
into in the monite-app
element as shown:
<monite-app
entity-id="ENTITY_ID"
api-url="https://api.sandbox.monite.com/v1"
basename="/"
>
<!-- Embed JSON into the monite-app element -->
<script slot="locale" type="application/json">
{
"code": "de-DE",
"messages": { "Counterparts": "Suppliers" }
}
</script>
</monite-app>
Next steps
By completing this quick start, you have learned how to:
- Install and set up Monite Drop-in SDK.
- Localize it and change the default appearance.
To learn more about this integration, see Drop-in library.
Updated about 1 month ago